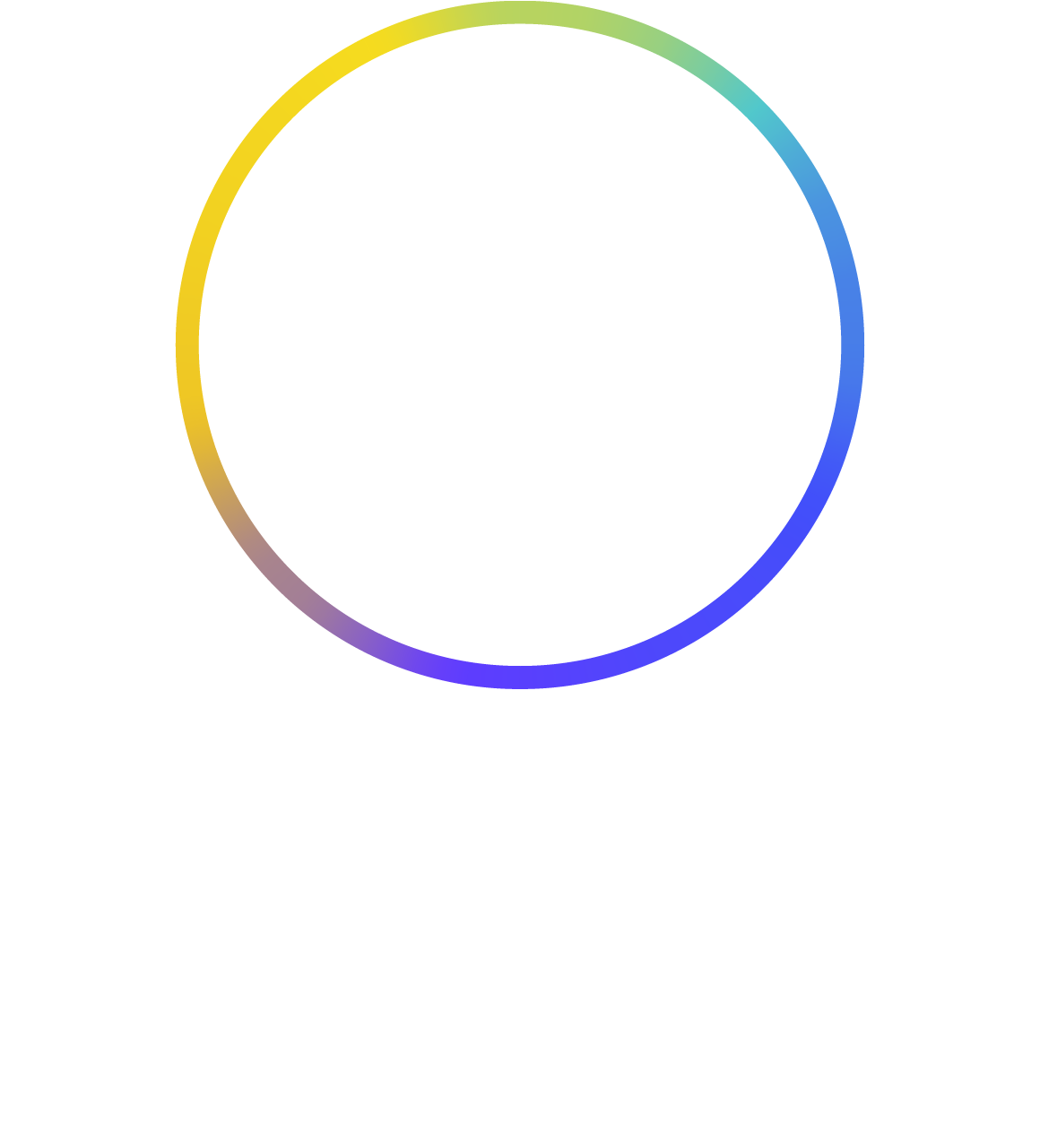
npm:
npm i @lavanet/lava-cosmjs
- create()
- new() ...init()
- new()...relay
const { Tendermint37Client } = require("@cosmjs/tendermint-rpc");
const { LavaCosmJsRPCClient } = require("@lavanet/lava-cosmjs");
async function getAbciInfo() {
//LavaCosmJsRPCClient is initialized upon calling create()
const client = await LavaCosmJsRPCClient.create({
// privatekey or badge must be supplied
badge: {
badgeServerAddress: "https://badges.lavanet.xyz",
projectId: "//" //login to gateway.lavanet.xyz to get a projectId!
},
chainIds: "LAV1",
lavaChainId: "lava-testnet-2",
geolocation: "1", //optional
})
const client = await Tendermint37Client.create(client);
console.log(client.abciInfo());
}
const { Tendermint37Client } = require("@cosmjs/tendermint-rpc");
const { LavaCosmJsRPCClient } = require("@lavanet/lava-cosmjs");
async function getAbciInfo() {
//LavaCosmJsRPCClient is initialized by calling init() after new()
const client = new LavaCosmJsRPCClient({
// privatekey or badge must be supplied
badge: {
badgeServerAddress: "https://badges.lavanet.xyz",
projectId: "//" //login to gateway.lavanet.xyz to get a projectId!
},
chainIds: "LAV1",
lavaChainId: "lava-testnet-2",
geolocation: "1", //optional
})
//client is initialized at the following call
await client.init()
const cosmJS = Tendermint37Client.create(client);
console.log(cosmJS.abciInfo());
}
const { Tendermint37Client } = require("@cosmjs/tendermint-rpc");
const { LavaCosmJsRPCClient } = require("@lavanet/lava-cosmjs");
async function getAbciInfo() {
//LavaCosmJsRPCClient is initialized at first relay
const client = new LavaCosmJsRPCClient({
// privatekey or badge must be supplied
badge: {
badgeServerAddress: "https://badges.lavanet.xyz",
projectId: "//" //login to gateway.lavanet.xyz to get a projectId!
},
chainIds: "LAV1",
lavaChainId: "lava-testnet-2",
geolocation: "1", //optional
})
const client = Tendermint37Client.create(client);
//client is initialized at the following call
console.log(client.abciInfo());
}
❓ Looking for more examples? Check out the examples folder on our repository.